How To Use Filter Javascript
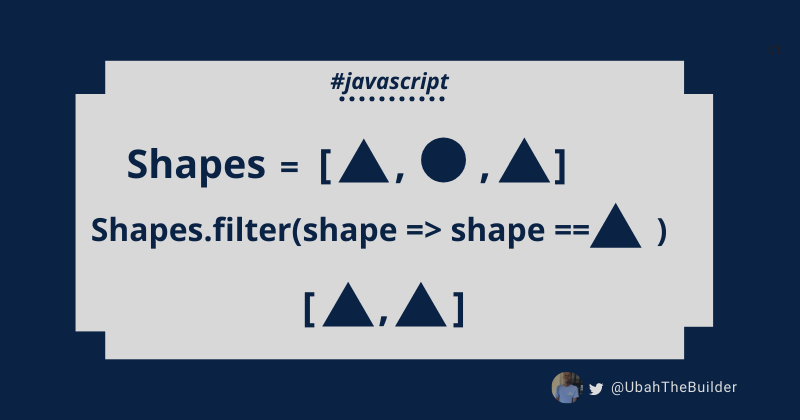
The Array.filter()
method is arguably the nearly of import and widely used method for iterating over an array in JavaScript.
The way the filter()
method works is very simple. It entails filtering out ane or more items (a subset) from a larger collection of items (a superset) based on some condition/preference.
Nosotros all do this everyday, whether we're reading, choosing friends or our spouse, choosing what movie to sentinel, and then on.
The JavaScript Assortment.filter()
Method
The filter()
method takes in a callback function and calls that role for every detail it iterates over inside the target array. The callback function can take in the post-obit parameters:
-
currentItem
: This is the element in the array which is currently being iterated over. -
index
: This is the alphabetize position of thecurrentItem
within the array. -
assortment
: This represents the target array along with all its items.
The filter method creates a new array and returns all of the items which pass the condition specified in the callback.
How to Apply the filter()
Method in JavaScript
In the post-obit examples, I will demonstrate how you tin utilise the filter()
method to filter items from an array in JavaScript.
filter()
Example one: How to filter items out of an array
In this instance, we filter out every person who is a toddler (whose age falls between 0 and iv ).
permit people = [ {proper name: "aaron",age: 65}, {name: "beth",historic period: ii}, {name: "cara",age: 13}, {proper name: "daniel",historic period: 3}, {name: "ella",age: 25}, {name: "fin",age: 1}, {proper name: "george",age: 43}, ] let toddlers = people.filter(person => person.age <= 3) panel.log(toddlers) /* [{ age: 2, proper name: "beth" }, { historic period: 3, name: "daniel" }, { age: one, name: "fin" }] */
filter()
Example 2: How to filter out items based on a particular holding
In this instance, we will just exist filtering out the team members who are developers.
let team = [ { name: "aaron", position: "programmer" }, { name: "beth", position: "ui designer" }, { name: "cara", position: "developer" }, { name: "daniel", position: "content manager" }, { proper name: "ella", position: "cto" }, { name: "fin", position: "backend engineer" }, { name: "george", position: "developer" }, ] let developers = squad.filter(member => fellow member.position == "developer") console.log(developers) /* [{ name: "aaron", position: "developer" }, { name: "cara", position: "developer" }, { proper name: "george", position: "programmer" }] */
In the above example, we filtered out the developers. But what if we want to filter out every member who is not a developer instead?
We could do this:
allow squad = [ { name: "aaron", position: "developer" }, { name: "beth", position: "ui designer" }, { proper noun: "cara", position: "developer" }, { name: "daniel", position: "content managing director" }, { name: "ella", position: "cto" }, { name: "fin", position: "backend engineer" }, { proper noun: "george", position: "developer" }, ] let nondevelopers = team.filter(member => member.position !== "developer") panel.log(nondevelopers) /* [ { name: "beth", position: "ui designer" }, { name: "daniel", position: "content manager" }, { name: "ella", position: "cto" }, { name: "fin", position: "backend engineer" } ] */
filter()
Case 3: How to access the index belongings
This is a competition. In this contest, there are three winners. The first will get the aureate medal, the 2nd volition get silver, and the third, statuary.
Past using filter
and accessing the alphabetize
property of every detail on each iteration, we can filter out each of the 3 winners into dissimilar variables.
allow winners = ["Anna", "Beth", "Cara"] let gold = winners.filter((winner, index) => index == 0) let silvery = winners.filter((winner, index) => alphabetize == 1) let statuary = winners.filter((winner, index) => alphabetize == 2) console.log(Aureate winner: ${gold}, Silver Winner: ${silver}, Bronze Winner: ${bronze}) // "Gold winner: Anna, Argent Winner: Beth, Statuary Winner: Cara"
filter()
Example iv: How to use the array parameter
Ane of the most common uses of the third parameter (assortment) is to inspect the state of the array beingness iterated over. For example, nosotros tin can check to run into if there is another item left in the array. Depending on the outcome, we tin specify that different things should happen.
In this example, nosotros are going to define an assortment of four people. However, since there can only be three winners, the fourth person in the list volition take to be discounted.
To be able to do this, we need to get data about the target array on every iteration.
let competitors = ["Anna", "Beth", "Cara", "David"] function displayWinners(proper noun, index, array) { let isNextItem = index + i < array.length ? true : false if (isNextItem) { panel.log(`The No${index+ane} winner is ${proper noun}.`); } else { panel.log(`Deplorable, ${name} is not one of the winners.`) } } competitors.filter((name, alphabetize, array) => displayWinners(proper name, index, array)) /* "The No1 winner is Anna." "The No2 winner is Beth." "The No3 winner is Cara." "Sorry, David is non one of the winners." */

How to Use the Context Object
In addition to the callback function, the filter()
method tin also have in a context object.
filter(callbackfn, contextobj)
This object can then be referred to from within the callback function using the this
keyword reference.
filter()
Case 5: How to access the context object with this
This is going to be similar to example 1
. Nosotros are going to be filtering out every person who falls between the ages of 13 and 19 (teenagers).
Nonetheless, we will not be hardcoding the values within of the callback function. Instead, nosotros will define these values 13 and 19 as properties inside the range
object, which we will subsequently pass intofilter
every bit the context object (second parameter).
let people = [ {proper name: "aaron", age: 65}, {name: "beth", historic period: 15}, {name: "cara", age: xiii}, {name: "daniel", age: 3}, {name: "ella", historic period: 25}, {proper name: "fin", age: sixteen}, {proper name: "george", age: xviii}, ] let range = { lower: 13, upper: 16 } let teenagers = people.filter(function(person) { return person.age >= this.lower && person.age <= this.upper; }, range) console.log(teenagers) /* [{ historic period: fifteen, name: "beth" }, { age: xiii, proper name: "cara" }, { age: 16, name: "fin" }] */
We passed the range
object as a second argument to filter()
. At that betoken, it became our context object. Consequently, we were able to access our upper and lower ranges in our callback office with the this.upper
and this.lower
reference respectively.
Wrapping Up
The filter()
array method filters out item(south) which friction match the callback expression and returns them.
In add-on to the callback role, the filter
method can besides take in a context object every bit the second argument. This will enable you admission any of its properties from the callback function using this
.
I hope you got something useful from this article.
I f you desire to learn more than about Web Development, experience free to visit my Web log .
Thank you lot for reading and see you soon.
P/South : If you lot are learning JavaScript, I created an eBook which teaches 50 topics in JavaScript with hand-drawn digital notes. Check information technology out here.
Learn to code for gratuitous. freeCodeCamp'southward open source curriculum has helped more than than forty,000 people get jobs as developers. Go started
How To Use Filter Javascript,
Source: https://www.freecodecamp.org/news/javascript-array-filter-tutorial-how-to-iterate-through-elements-in-an-array/
Posted by: marshallbelank.blogspot.com
0 Response to "How To Use Filter Javascript"
Post a Comment